Although creating 3D models using Blender, Cinema4D, or (insert favourite 3D modeller here) is fun, programming 3D models is even .. funnerer. Here’s a few bits of info about this…
General Programming Languages
We can program 3D geometric meshes using just about any general programming language available. There are a number of libraries available for c++, for instance, that offer an API for creating, accessing, and modifying a mesh. Some examples are VCGLib (template joy!), OpenMesh (the simplest), and CGAL (heavy, has bindings for other languages.)
The generality of a programming language like C++ means that programming a mesh is more tedious than it should be. For example, it wouldn’t be out of the ordinary to see code like this:
Mesh m; std::vector& verts = m.verts(); for(std::vector::iterator it = verts.begin();it!=verts.end();it++){ Vertex& v = *it; std::vector neighbours = v.neighbours(); float m = 0; for(std::vector::iterator nit = neighbours.begin();nit!=neighbours.end();nit++){ m += nit->data(); } v.setData(m/neighbours.size()); }
Erhk! A lot of power of programming comes from abstraction, so we can implement all the things we want and lock them away into a neat API, or scripting language. Fugu is an implementation of this idea and provides a user a few methods and functions for manipulating a mesh. On top of that, it uses the Lua scripting language, which has a friendlier syntax, and can be executed much quicker than compiled languages. The above program in Lua looks something like this:
m = Mesh() foreach(vertices(m), function(v) v.data = 0 local neighbours = nearby(v,1) foreach(neighbours, function(n) v.data = n.data end) v.data = v.data/#neighbours end)
Lua is popular in game development because it is small, fast and easy to embed. The most popular example World of Warcraft, where it is used customise the UI. Perhaps most relevant to this discussion, is that Lua has also been used in a real-time multimedia programming environment, LuaAV (link). From their github page…
LuaAV is a real-time audiovisual scripting environment based around the Lua scripting language and a collection of libraries for sound, graphics, and media protocols. The goal of LuaAV is to serve as a computational platform for creative exploration that is both fluid and generative.
Here’s another similar Lua-based multimedia environment (I think, it’s in spanish so I have no idea what it is…!)
Another approach to mesh programming is to come up with a new language — specifically for the creation and manipulation of meshes. Vertex-vertex systems and GML are two such languages..
Vertex-Vertex Systems
In his dissertation, On Vertex-Vertex Systems and Their Use in Geometric and Biological Modelling (link), Colin Smith introduced a neat “programming language” for operating on meshes, called Vertex-Vertex Systems, or VV, for short. The primary data structure in this language is the mesh, a collection of vertices and their local topology (a list of ordered neighbours). A key property of VV systems is that the elements of the mesh aren’t stored as an indexable list, but rather as a collection of vertices connected locally with a specific structure. Operations, such as insert and remove are available for modifying the mesh.
Smith’s dissertation is interesting to because he offers several examples of VV models, from different domains. For example, he implements a variety of smooth subdivision algorithms, demonstrating the terseness and readability of VV over conventional programming languages. He also demonstrates a number of biological models of growth. These are quite natural to model with VV.
Unfortunately, like most PhD projects, VV seems to be lost in the void (Smith is now working at a big animation studio). I have no doubt that a system like this would greatly improve the robustness and clarity of mesh-based programs, such as 3D modelling programs, generative software, and the like. (Factors like compactness of data structure and speed would have to be considered though.)
Generative Modelling Language
The generative modelling language, or GML (link), is a mesh-building language derived from Postscript. A GML program describes the construction of an object, through the concatenation of many operations. It is postfix (read: backwards), which gives it a unique charm (and makes the implementation of an interpreter trivial!).
In order to build/modify a mesh you need a way to access different parts of it. In GML you always have a handle on some part of the mesh. Each operation either transforms the mesh and gives you a new handle, or changes the position of the handle on the mesh. So for instance, if the handle was just a face of the mesh, and you executed the extrude operation, your new handle might be the face at the end of the extrusion. This assumption means that some things (like concatenating extrusions) are really easy … and other things are a little more tricky.
GML is a pretty nifty language and is targetted primarily towards “rigid” meshes, like buildings, hubcaps, and … chairs.
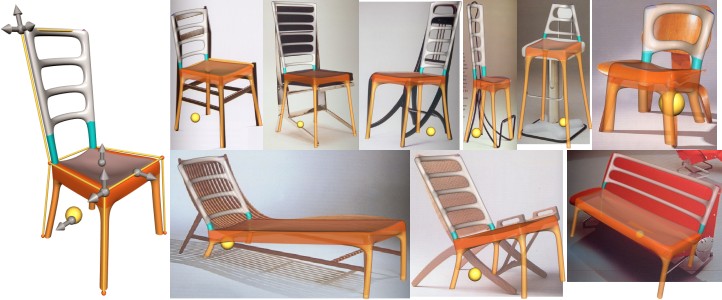
The thing about GML is that, although it is a solid system with strictly specified semantics and a rich set of geometric operations, the tools for making GML models are … not good. It’s all ActiveX this, and ActiveX that. This seems like a mistake — the GML guys should instead have written a specific library, in c++, that could then be used in different applications, e.g., as a plugin for a Autocad or whatever. This kind of illustrates why any platform-specific technology should never be used — though as I write this I’m about to develop some Obj-C stuff (but what’s humanity without hypocrisy!)
I wouldn’t be so concerned about the tools if GML could be written by a human. Instead, it’s a human-readable but only-machine-understandable format (like XML). Want to add two numbers, here’s the program … “2 5 +”. Postfix is only awesome from a theoretical perspective, and a simplicity-of-implementation perspective. But as the interpreter will only be written a few times and GML programs many times, why should the simplicity be on the developer’s side? As an output format, GML seems is one of the more interesting ones, we just need some decent tools to work with it.
I should also give GSculpt a mention (link), it is a 3D modeller that stores the entire history of the modelling process. This history can then be re-executed to show how the model was built, or indeed modified to produce variants of the model. Gsculpt supports an equally huge number of operations as GML, but unfortunately has a dated and clumsy UI.